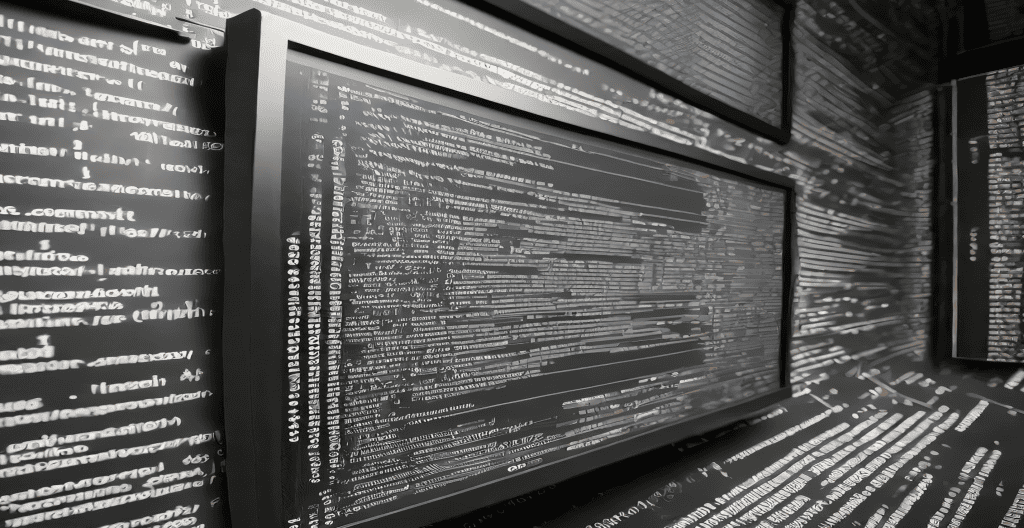
A shell is a command-line interface (CLI) that provides a user with a way to interact with an operating system, execute commands, and manage the system. Shells are an essential component of Unix-like operating systems, including Linux, macOS, and various Unix distributions. Bash, sh, c shell, Zsh are different types of shell. These shells function as an intermediary between the user and the operating system, allowing users to perform various tasks and operations.
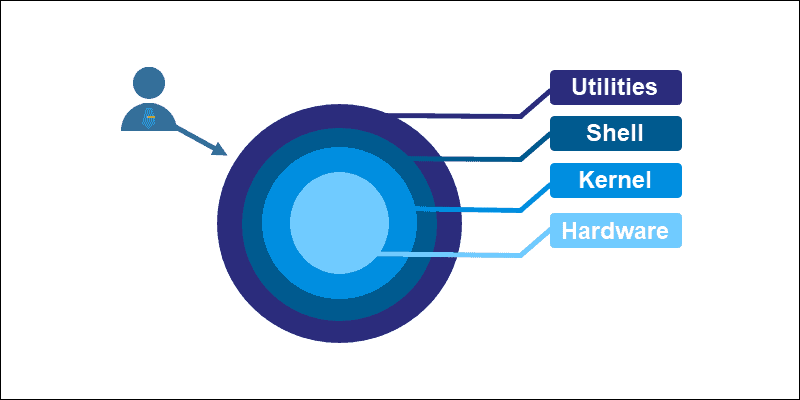
A shell script is a computer program designed to be run by the Unix/Linux shell. Linux provides a variety of shells with distinct functionality to tackle a wide range of issues. As Unix development went on, the shells frequently appropriated functionality from one another. Additionally, each shell offers specific capabilities for scripting and automation, making it a versatile choice for system administrators and developers.
In this article you will learn:
- Different types of Shell
- What is Bash(Bourne-Again SHell)?
- What is Bash Scripting and the common elements of Bash script.
- How to create a simple bash script?
- Advantages of Bash Scripting
Here are some of the most commonly used Linux shells:
- Bourne Shell (sh)
- C Shell (csh)
- Zsh (Z Shell)
- Fish (Friendly Interactive SHell)
- Dash (Debian Almquist Shell)
- Ksh (KornShell)
- Tcsh (TENEX C Shell)
- Ash (Almquist Shell)
- Bash (Bourne-Again SHell)
You can determine your shell type using the ps
command.
Bash (Bourne-Again SHell)
The Bash shell is one of the most popular and widely used command shells on Unix-like operating systems, including Linux. Furthermore, it is an enhanced and extended version of the original Bourne Shell, which was initially created by Stephen Bourne at AT&T Bell Labs in the late 1970s..
The development of Bash began in the late 1980s when Brian Fox started working on a free software alternative to the Bourne Shell. His goal was to create a shell that was compatible with the Bourne Shell while adding new features and improvements. Bash introduced several features, such as command-line editing, history tracking, improved scripting capabilities, and more. These enhancements marked a significant advancement over the original Bourne Shell. Furthermore, these additions not only improved user interaction but also empowered scriptwriters and system administrators with a more versatile and user-friendly environment.
Bash’s compatibility with the Bourne Shell means that many scripts and commands written for the Bourne Shell can seamlessly run in Bash without modification. Furthermore, this backward compatibility has significantly contributed to its widespread use.
Bash quickly gained popularity in the Unix and Linux communities and became the default shell on many Linux distributions due to its power and versatility.
The shell name shortens to bash, and the location is /bin/bash.
Bash Scripting
Bash scripting allows you to automate tasks and perform various operations using the Bash shell. Here, I’ll provide a brief overview of Bash scripting and some common elements you’ll encounter when writing Bash scripts.
1. Shebang Line: A Bash script typically starts with a shebang line that specifies the path to the Bash interpreter. It looks like this:
#!/bin/bash
for this we need to find out where is Bash interpreter located. For this we can use the command :
$ which bash
/bin/bash
This command reveals that the Bash shell is stored in /bin/bash
.
2. Comments: You can include comments in your script to provide explanations or documentation. Bash comments start with #
.
# This is a comment
3. Variables: You can declare and use variables in Bash. Variables are typically assigned values using '=
‘ .
my_variable="Hello, World!"
4. User Input: You can prompt the user for input and store it in a variable using the read
command.
echo "Please enter your name: "
read username
echo "Hello, $username!"
5. Control Structures: Bash supports conditional statements (if-else), loops (for, while), and case statements for more complex script flow control.
if [ "$value" -eq 5 ]; then
echo "Value is 5."
else
echo "Value is not 5."
fi
6. Functions: You can define functions in Bash to encapsulate reusable code.
my_function() {
echo "This is a function."
}
my_function
Here’s a Simple Bash Shell Script that prompts the user for their name, greets them, and then prints the current date and time:
[root@ervintest ~]# vi ./example.sh
Copy and paste the script into the text editor.
#!/bin/bash
# Prompt the user for their name
echo "Hello! Please enter your name: "
read username
# Greet the user
echo "Hello, $username! Welcome to our simple Bash script."
# Print the current date and time
current_date=$(date)
echo "The current date and time is: $current_date"
Save the script with a .sh
extension
To make the script executable, you can run the following command in the terminal. Once it’s executable, you can simply execute it from the terminal by using ‘./’ followed by the script’s name.
[root@ervintest ~]# chmod +x example.sh
[root@ervintest ~]# ./example.sh
The script will ask the user for their name, greet them, and then display the current date and time.

Kindly refer more examples in https://linuxhint.com/30_bash_script_examples/
Advantages of Bash Script
- Compatibility: Bash is backward-compatible with sh. This means that most sh scripts can be executed by Bash without modification. Therefore, you can write scripts in a more feature-rich language (Bash) while ensuring compatibility with systems that use sh as the default shell.
- Improved Features:
- Interactive Features: Bash provides improved interactive features like command history, tab-completion, and customizable prompts, making it a more user-friendly shell for both scripting and manual interaction.
- Advanced String Handling: Bash offers advanced string manipulation features that make text processing in scripts more efficient and flexible.
- Better Scripting Capabilities:
- Arrays: Bash supports arrays, making it easier to work with lists of items.
- Functions: Bash supports the creation of functions, allowing you to encapsulate and reuse code more effectively.
- Advanced Control Structures: Bash offers more powerful control structures, including
for
,while
, anduntil
loops, and more advanced conditional statements withif
andcase
. - Arithmetic Operations: Bash allows for arithmetic operations directly within scripts without relying on external commands.
- Improved Command Substitution: Bash provides more versatile command substitution using backticks (`) and
$()
for embedding command output within scripts. - Process Substitution: Bash supports process substitution (e.g.,
<(command)
and>(command)
) for working with the input and output of commands as if they were files. This is useful for complex data manipulation and working with multiple processes. - Enhanced Pattern Matching: Bash supports advanced pattern matching with regular expressions using
=~
and globbing. - Extended Parameter Expansion: Bash offers extended parameter expansion options, enabling more advanced text manipulation.
- Job Control: Bash includes built-in job control for managing processes and background jobs, which is not available in sh.
- More Robust Error Handling: Bash provides better error handling mechanisms, including the
trap
command, to manage signals and errors in scripts more effectively. - Compatibility Across Unix-like Systems: Bash is available on virtually all Unix-like systems and is often the default shell, making it a more portable choice for scripting across different platforms.
In summary, Bash scripting provides a more powerful and user-friendly scripting environment. Moreover, it offers a wide range of features that greatly enhance script development, maintenance, and execution. Furthermore, Bash’s backward compatibility with sh further reinforces its versatility, making it a safer and more practical choice for most scripting tasks. This is because scripts written in Bash can generally run seamlessly on systems that use sh as the default shell.